How to Write to the STM32 Flash Memory Using HAL
You first need to prepare the flash memory for writing
3 min. read
Published on
Read our disclosure page to find out how can you help Windows Report sustain the editorial team. Read more
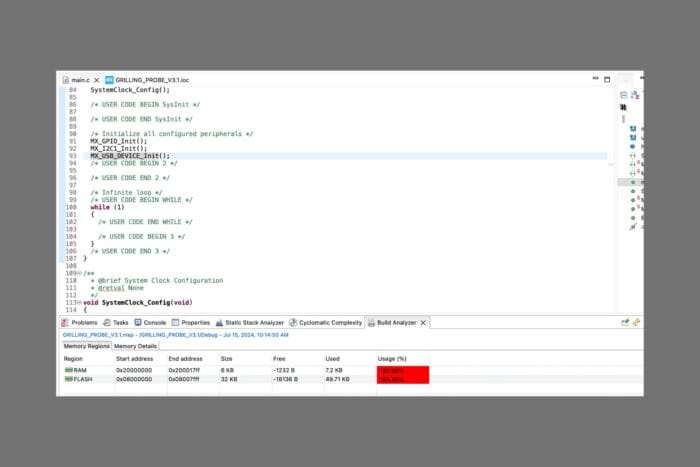
If you decided on a STM32 flash memory HAL approach, here’s the basics on how to program your microcontroller easily. The Hardware Abstraction Layer (HAL) should be pretty simple to set up using a few examples below.
How do I write on STM32 flash memory using HAL?
1. Prepare the flash memory for writing
- Include necessary headers:
#include "stm32f4xx_hal.h"
- Unlock the flash memory: Before you can write to the flash memory, you need to unlock it by using the following command:
HAL_FLASH_Unlock();
- Erase the Flash Memory: You need to erase the sector of the flash memory where you want to write data. This is done using the following HAL_FLASHEx_Erase function:
FLASH_EraseInitTypeDef EraseInitStruct; uint32_t SectorError; EraseInitStruct.TypeErase = FLASH_TYPEERASE_SECTORS; EraseInitStruct.Sector = FLASH_SECTOR_2; // Choose the sector you want to erase EraseInitStruct.NbSectors = 1; EraseInitStruct.VoltageRange = FLASH_VOLTAGE_RANGE_3; if (HAL_FLASHEx_Erase(&EraseInitStruct, &SectorError) != HAL_OK) { // Handle the error }
2. Write data for the flash memory
- Use the HAL_FLASH_Program function to write data to the flash memory. You can write data in bytes, half-words, words, or double words.
uint32_t Address = 0x08008000; // Starting address in flash memory uint32_t Data = 0x12345678; // Data to be written if (HAL_FLASH_Program(FLASH_TYPEPROGRAM_WORD, Address, Data) != HAL_OK) { // Handle the error }
- Lock the flash memory – After writing the data, lock the flash memory to prevent accidental writes:
HAL_FLASH_Lock();
Example code on STM32 flash memory HAL coding
Here’s a complete example that combines all the steps:
#include "stm32f4xx_hal.h"
void Write_Flash(uint32_t Address, uint32_t Data) {
// Unlock the Flash
HAL_FLASH_Unlock();
// Erase the Flash sector
FLASH_EraseInitTypeDef EraseInitStruct;
uint32_t SectorError;
EraseInitStruct.TypeErase = FLASH_TYPEERASE_SECTORS;
EraseInitStruct.Sector = FLASH_SECTOR_2;
EraseInitStruct.NbSectors = 1;
EraseInitStruct.VoltageRange = FLASH_VOLTAGE_RANGE_3;
if (HAL_FLASHEx_Erase(&EraseInitStruct, &SectorError) != HAL_OK) {
// Handle the error
}
// Program the Flash memory
if (HAL_FLASH_Program(FLASH_TYPEPROGRAM_WORD, Address, Data) != HAL_OK) {
// Handle the error
}
// Lock the Flash
HAL_FLASH_Lock();
}
int main(void) {
HAL_Init();
Write_Flash(0x08008000, 0x12345678);
while (1) {
// Main loop
}
}
This example demonstrates how to unlock, erase, write to, and lock the flash memory of an STM32 microcontroller using HAL functions. We hope that our guide helped you with STM32 flash memory HAL coding.
If you have any issues, refer to out guide to fix the HAL INITIALIZATION FAILED error.
If you have any questions or suggestions, don’t hesitate to write it down in the comments section below.
User forum
0 messages